Hi! The following video crashes the player (with width
and height
constants adjusted to 1024
and 576
) quite consistently:
https://user-images.githubusercontent.com/245089/197802318-9dbc7afd-8238-4983-8154-0f61bfb5b26d.mp4
It looks like a heap corruption (most likely a buffer overflow somewhere) and happens on Windows and Linux. When it does not crash, one can also see some corrupted pixels at the top of the screen. See screenshot:
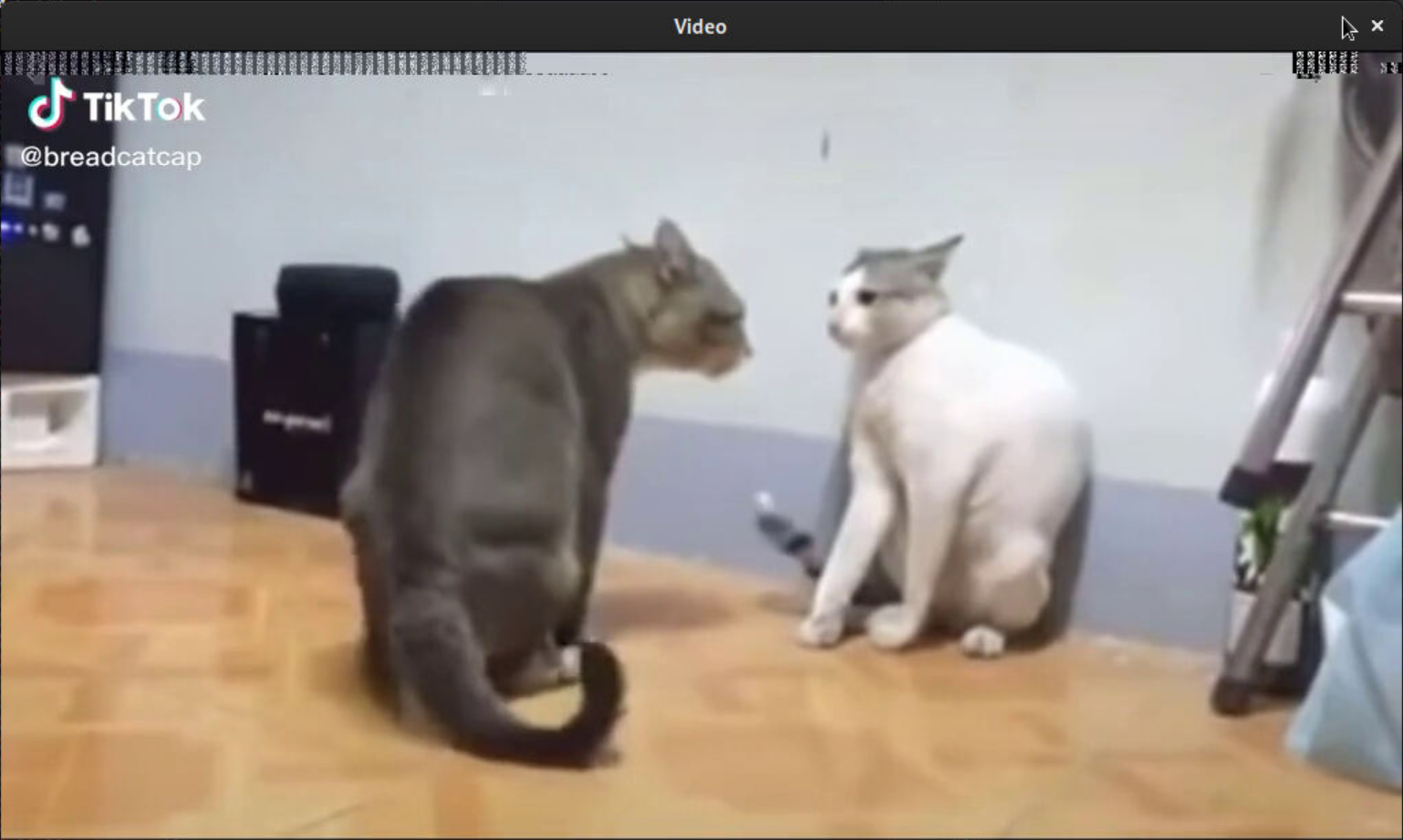
The ffplayer
utility plays the video without visible issues.
Linux crash message looks like this:
corrupted size vs. prev_size
Thread 8 "player" received signal SIGABRT, Aborted.
The call stack looks like this in GDB/Linux:
#0 __pthread_kill_implementation (threadid=<optimized out>, signo=signo@entry=0x6, no_tid=no_tid@entry=0x0) at ./nptl/pthread_kill.c:44
#1 0x00007ffff5e8989f in __pthread_kill_internal (signo=0x6, threadid=<optimized out>) at ./nptl/pthread_kill.c:78
#2 0x00007ffff5e3da52 in __GI_raise (sig=sig@entry=0x6) at ../sysdeps/posix/raise.c:26
#3 0x00007ffff5e28469 in __GI_abort () at ./stdlib/abort.c:79
#4 0x00007ffff5e7dc18 in __libc_message (action=action@entry=do_abort, fmt=fmt@entry=0x7ffff5fb4689 "%s\n") at ../sysdeps/posix/libc_fatal.c:155
#5 0x00007ffff5e9355a in malloc_printerr (str=str@entry=0x7ffff5fb2161 "corrupted size vs. prev_size") at ./malloc/malloc.c:5531
#6 0x00007ffff5e93eb6 in unlink_chunk (p=<optimized out>, av=0x7fffa4000020) at ./malloc/malloc.c:1626
#7 0x00007ffff5e94040 in malloc_consolidate (av=av@entry=0x7fffa4000020) at ./malloc/malloc.c:4663
#8 0x00007ffff5e95d88 in _int_malloc (av=av@entry=0x7fffa4000020, bytes=bytes@entry=0xa10) at ./malloc/malloc.c:3848
#9 0x00007ffff5e96e9f in _int_memalign (av=av@entry=0x7fffa4000020, alignment=alignment@entry=0x40, bytes=bytes@entry=0x9a7) at ./malloc/malloc.c:4851
#10 0x00007ffff5e9752a in _mid_memalign (alignment=alignment@entry=0x40, bytes=bytes@entry=0x9a7, address=<optimized out>) at ./malloc/malloc.c:3453
#11 0x00007ffff5e98a2f in __posix_memalign (size=0x9a7, alignment=0x40, memptr=0x7fffbe1c03b0) at ./malloc/malloc.c:5557
#12 __posix_memalign (memptr=0x7fffbe1c03b0, alignment=0x40, size=0x9a7) at ./malloc/malloc.c:5541
#13 0x00007ffff7e05aad in av_malloc () at /lib/x86_64-linux-gnu/libavutil.so.57
#14 0x00007ffff7dd2d15 in av_buffer_alloc () at /lib/x86_64-linux-gnu/libavutil.so.57
#15 0x00007ffff7dd2d8e in av_buffer_allocz () at /lib/x86_64-linux-gnu/libavutil.so.57
#16 0x00007ffff7dd3656 in av_buffer_pool_get () at /lib/x86_64-linux-gnu/libavutil.so.57
#17 0x00007ffff6795da4 in () at /lib/x86_64-linux-gnu/libavcodec.so.59
#18 0x00007ffff679a148 in () at /lib/x86_64-linux-gnu/libavcodec.so.59
#19 0x00007ffff679eca5 in () at /lib/x86_64-linux-gnu/libavcodec.so.59
#20 0x00007ffff667e841 in () at /lib/x86_64-linux-gnu/libavcodec.so.59
#21 0x00007ffff667f4c0 in avcodec_send_packet () at /lib/x86_64-linux-gnu/libavcodec.so.59
#22 0x0000000000573c3b in _cgo_c6952d519846_Cfunc_avcodec_send_packet (v=0xc000233628) at /tmp/go-build/cgo-gcc-prolog:276
#23 0x00000000004722a4 in runtime.asmcgocall () at /usr/lib/go-1.19/src/runtime/asm_amd64.s:844
#24 0x0000000000000001 in ()
#25 0x0000000000443900 in runtime.fatal at /usr/lib/go-1.19/src/runtime/panic.go:1062
#26 0x0000000000470429 in runtime.systemstack () at /usr/lib/go-1.19/src/runtime/asm_amd64.s:492
#27 0x0000000000000000 in ()